Extending the Android Notepad tutorial (Notepad v4)
In order to develop my understanding of Android and the Notepad tutorial I decided to extend it, and correct some bugs. I first created a copy of the Notepad3solution tutorial directory named NotepadV4 and created an Eclipse Project called NotepadV4 from this source code. The detailed steps to accomplish this within Eclipse were given in my previous post. Once you have the project up on running on the emulator it will look like this.
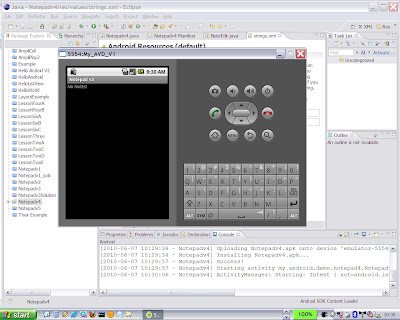
The first change is to correct the application name shown in the ListView. Changing the application name is easy using the XML editor provided within the ADT Eclipse plugin. Simply update the app_name string within strings.xml using the editors resources tab as shown below.
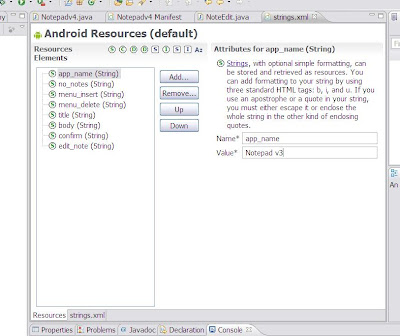
The next change is to improve the No Notes! message. Due to a minor bug within Notepad3solution, this is currently defined within notes_list.xml in the following:
Change the final line to:
android:text="@string/no_notes"/>
and update the no_notes string within strings.xml to an appropriate message using the XML editor. I chose “To add a note press Menu”
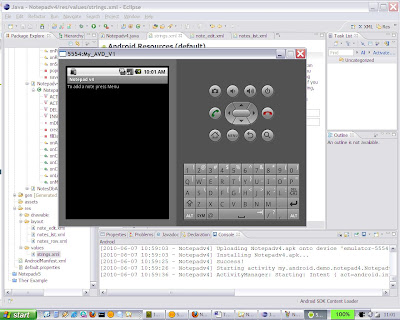
Now lets fix a more serious bug. Starting with no notes present try creating three notes. The first has a title of “1” and a body of “Body 1”, the second has a null title and a body of “Body 2”, the third with a title of “3” and a body of “Body 3”. You will see the following:
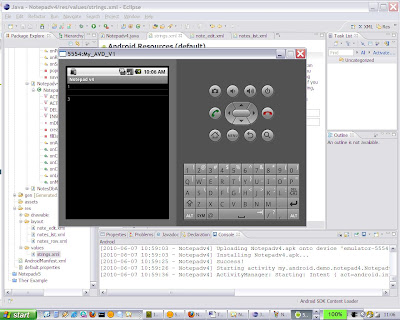
You will see that all three notes are there. Now try deleting Note 2 … as the title is null it is currently impossible to select the note using the context menu, and hence to delete it. To correct this add the following two lines toward the end of the layout file notes_row.xml
android:padding="10dp"
android:textSize="16sp"
This puts some padding around the text in each notes row, which allows us to select a note even if it has a null title. It also increases the font size.
Now lets extend the context menu so it can be used to add, edit or delete a note. Firstly near the beginning of NotepadV4.java amend the constants defining the position of the menu items in the context menu as follows:
private static final int INSERT_ID = Menu.FIRST;
private static final int EDIT_ID = Menu.FIRST + 1;
private static final int DELETE_ID = EDIT_ID + 1;
Still with NotepadV4.java amend onCreateContextMenu to create the three context menu items as follows:
@Override
public void onCreateContextMenu(ContextMenu menu, View v,
ContextMenuInfo menuInfo) {
super.onCreateContextMenu(menu, v, menuInfo);
menu.add(0, INSERT_ID, 0, R.string.menu_insert);
menu.add(0, EDIT_ID, 0, R.string.menu_edit);
menu.add(0, DELETE_ID, 0, R.string.menu_delete);
}
Change OnContextItemSelected() to handle these three cases as follows:
@Override
public boolean onContextItemSelected(MenuItem item) {
AdapterContextMenuInfo info = (AdapterContextMenuInfo) item
.getMenuInfo();
switch (item.getItemId()) {
case INSERT_ID:
createNote();
return true;
case EDIT_ID:
editNote(info.id);
return true;
case DELETE_ID:
mDbHelper.deleteNote(info.id);
fillData();
return true;
}
return super.onContextItemSelected(item);
}
and finally create a new method editNote() by copying the createNote() method and amending it as follows:
private void editNote(long id) {
Intent i = new Intent(this, NoteEdit.class);
i.putExtra(NotesDbAdapter.KEY_ROWID, id);
startActivityForResult(i, ACTIVITY_EDIT);
}
When you try and run this project you will get an error
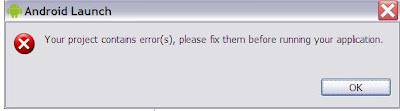
Here is the cause:
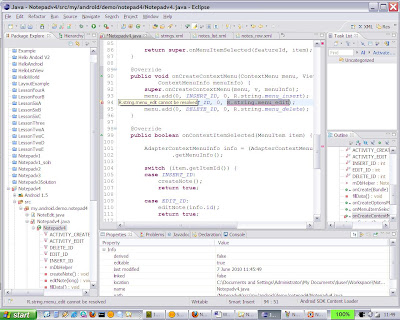
We have not defined a string called menu_edit within the strings.xml file. The string is currently called edit_note. Resolving this using the XML editor and running the application should give the following context menu:
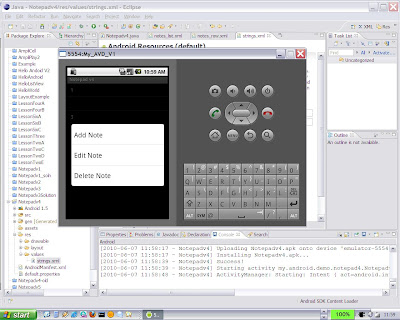
This version of the Notepad tutorial is definitely an improvement. In the next post we will look into another bug using Logcat.
The first change is to correct the application name shown in the ListView. Changing the application name is easy using the XML editor provided within the ADT Eclipse plugin. Simply update the app_name string within strings.xml using the editors resources tab as shown below.
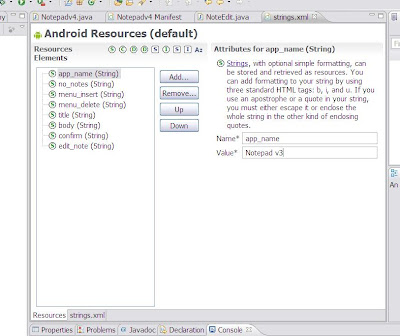
The next change is to improve the No Notes! message. Due to a minor bug within Notepad3solution, this is currently defined within notes_list.xml in the following:
textview id="@+id/android:empty" layout_width="wrap_content" layout_height="wrap_content" text="No Notes!"/>"'
Change the final line to:
android:text="@string/no_notes"/>
and update the no_notes string within strings.xml to an appropriate message using the XML editor. I chose “To add a note press Menu”
Now lets fix a more serious bug. Starting with no notes present try creating three notes. The first has a title of “1” and a body of “Body 1”, the second has a null title and a body of “Body 2”, the third with a title of “3” and a body of “Body 3”. You will see the following:
You will see that all three notes are there. Now try deleting Note 2 … as the title is null it is currently impossible to select the note using the context menu, and hence to delete it. To correct this add the following two lines toward the end of the layout file notes_row.xml
android:padding="10dp"
android:textSize="16sp"
This puts some padding around the text in each notes row, which allows us to select a note even if it has a null title. It also increases the font size.
Now lets extend the context menu so it can be used to add, edit or delete a note. Firstly near the beginning of NotepadV4.java amend the constants defining the position of the menu items in the context menu as follows:
private static final int INSERT_ID = Menu.FIRST;
private static final int EDIT_ID = Menu.FIRST + 1;
private static final int DELETE_ID = EDIT_ID + 1;
Still with NotepadV4.java amend onCreateContextMenu to create the three context menu items as follows:
@Override
public void onCreateContextMenu(ContextMenu menu, View v,
ContextMenuInfo menuInfo) {
super.onCreateContextMenu(menu, v, menuInfo);
menu.add(0, INSERT_ID, 0, R.string.menu_insert);
menu.add(0, EDIT_ID, 0, R.string.menu_edit);
menu.add(0, DELETE_ID, 0, R.string.menu_delete);
}
Change OnContextItemSelected() to handle these three cases as follows:
@Override
public boolean onContextItemSelected(MenuItem item) {
AdapterContextMenuInfo info = (AdapterContextMenuInfo) item
.getMenuInfo();
switch (item.getItemId()) {
case INSERT_ID:
createNote();
return true;
case EDIT_ID:
editNote(info.id);
return true;
case DELETE_ID:
mDbHelper.deleteNote(info.id);
fillData();
return true;
}
return super.onContextItemSelected(item);
}
and finally create a new method editNote() by copying the createNote() method and amending it as follows:
private void editNote(long id) {
Intent i = new Intent(this, NoteEdit.class);
i.putExtra(NotesDbAdapter.KEY_ROWID, id);
startActivityForResult(i, ACTIVITY_EDIT);
}
When you try and run this project you will get an error
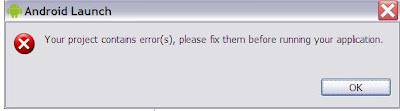
Here is the cause:
We have not defined a string called menu_edit within the strings.xml file. The string is currently called edit_note. Resolving this using the XML editor and running the application should give the following context menu:
This version of the Notepad tutorial is definitely an improvement. In the next post we will look into another bug using Logcat.
Comments
Post a Comment